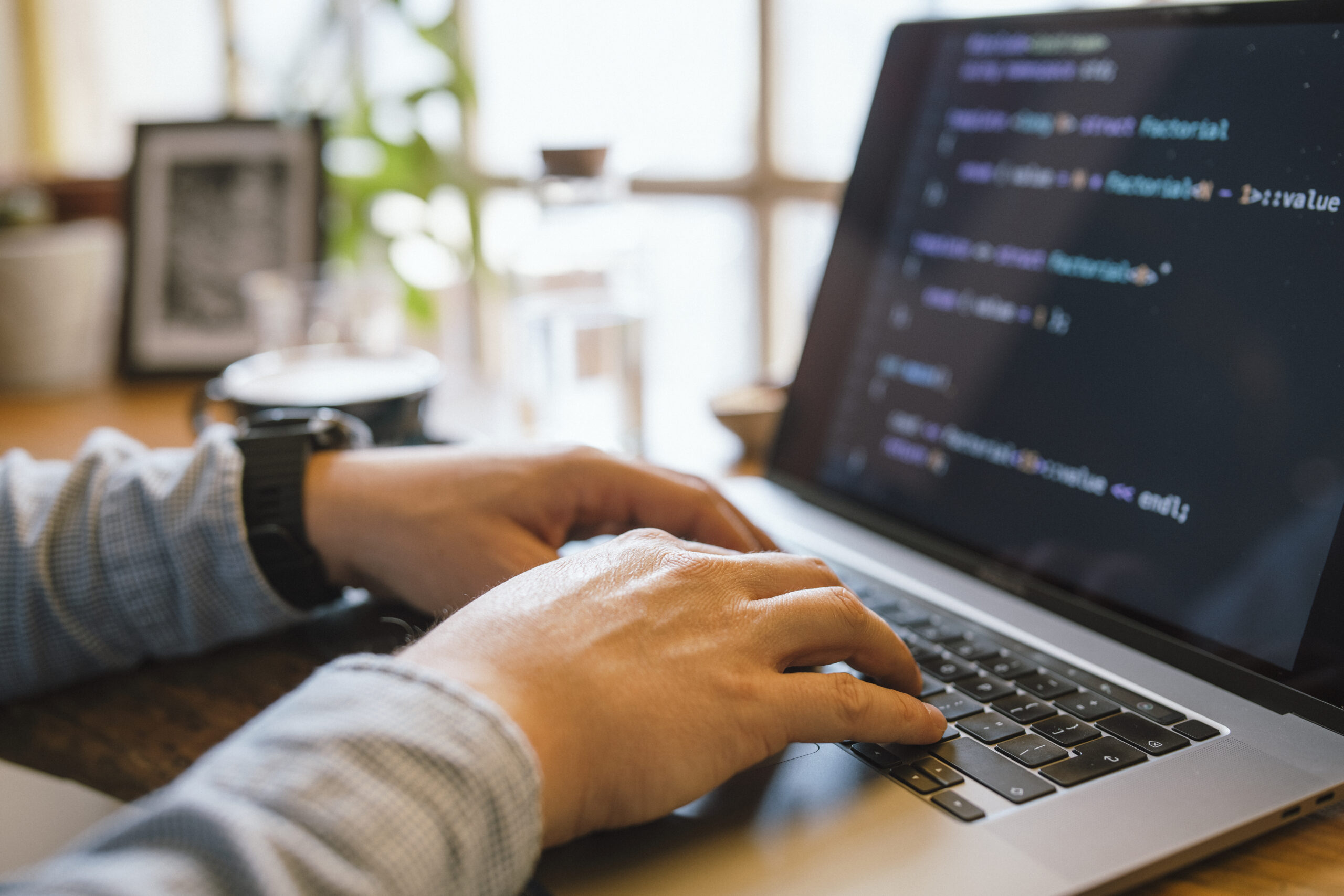
Debugging is One of the more crucial — still often ignored — skills within a developer’s toolkit. It is not pretty much correcting damaged code; it’s about comprehending how and why points go Completely wrong, and learning to think methodically to solve problems efficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of frustration and dramatically improve your efficiency. Here i will discuss quite a few procedures to assist developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several fastest approaches developers can elevate their debugging skills is by mastering the applications they use on a daily basis. When crafting code is just one Section of improvement, knowing ways to communicate with it efficiently for the duration of execution is equally important. Modern-day growth environments come Geared up with impressive debugging abilities — but numerous builders only scratch the floor of what these equipment can perform.
Take, such as, an Integrated Development Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources help you set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and even modify code about the fly. When used properly, they Permit you to observe exactly how your code behaves all through execution, and that is invaluable for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for entrance-stop builders. They let you inspect the DOM, check community requests, see serious-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can change disheartening UI problems into workable responsibilities.
For backend or method-stage builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Learning these resources could possibly have a steeper Studying curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be comfortable with Edition Handle devices like Git to be familiar with code history, locate the precise instant bugs were introduced, and isolate problematic modifications.
In the end, mastering your equipment signifies going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting so that when issues arise, you’re not misplaced at midnight. The better you realize your resources, the greater time it is possible to commit fixing the actual issue instead of fumbling via the procedure.
Reproduce the situation
Among the most important — and sometimes disregarded — measures in successful debugging is reproducing the situation. Ahead of jumping into the code or making guesses, builders need to have to create a steady atmosphere or situation where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a recreation of opportunity, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is gathering just as much context as you can. Ask issues like: What actions triggered The problem? Which setting was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it results in being to isolate the exact conditions underneath which the bug happens.
Once you’ve gathered adequate data, try to recreate the situation in your local natural environment. This could necessarily mean inputting precisely the same info, simulating identical consumer interactions, or mimicking process states. If the issue seems intermittently, consider composing automatic assessments that replicate the edge situations or point out transitions concerned. These assessments not only aid expose the issue and also stop regressions Sooner or later.
Sometimes, the issue could possibly be environment-precise — it might take place only on selected functioning techniques, browsers, or underneath individual configurations. Utilizing applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a step — it’s a attitude. It calls for tolerance, observation, in addition to a methodical approach. But when you can continually recreate the bug, you might be presently halfway to repairing it. That has a reproducible state of affairs, You may use your debugging applications more properly, take a look at probable fixes safely and securely, and converse additional Plainly with all your workforce or customers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders prosper.
Browse and Understand the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when anything goes Mistaken. As opposed to observing them as disheartening interruptions, builders must find out to treat mistake messages as immediate communications within the process. They typically let you know exactly what transpired, wherever it occurred, and occasionally even why it occurred — if you know how to interpret them.
Get started by looking at the message carefully As well as in total. Many developers, specially when below time pressure, look at the very first line and straight away start out producing assumptions. But deeper during the mistake stack or logs might lie the legitimate root result in. Don’t just duplicate and paste error messages into search engines like yahoo — browse and have an understanding of them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a particular file and line number? What module or purpose triggered it? These thoughts can guidebook your investigation and issue you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable styles, and Finding out to acknowledge these can dramatically increase your debugging method.
Some glitches are imprecise or generic, and in People situations, it’s very important to examine the context through which the error transpired. Look at connected log entries, enter values, and recent alterations during the codebase.
Don’t neglect compiler or linter warnings both. These normally precede bigger troubles and supply hints about prospective bugs.
Finally, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more rapidly, lower debugging time, and become a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, aiding you realize what’s taking place beneath the hood with no need to pause execution or move from the code line by line.
A very good logging system starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Information, WARN, ERROR, and Lethal. Use DEBUG for detailed diagnostic information during enhancement, Facts for general functions (like prosperous start out-ups), WARN for possible problems that don’t crack the applying, Mistake for real troubles, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Too much logging can obscure important messages and slow down your system. Deal with critical activities, state variations, input/output values, and critical conclusion details as part of your code.
Format your log messages Evidently and constantly. Include context, which include timestamps, request IDs, and performance names, so it’s simpler to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all with out halting the program. They’re In particular beneficial in generation environments exactly where stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Using a perfectly-believed-out logging tactic, you can decrease the time it will require to spot difficulties, acquire deeper visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Believe Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To proficiently detect and repair bugs, developers have to method the method just like a detective resolving a secret. This mindset assists break down intricate difficulties into workable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or overall performance problems. Much like a detective surveys a criminal offense scene, acquire as much pertinent details as it is possible to with no leaping to conclusions. Use logs, test situations, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Upcoming, sort hypotheses. Question your self: What might be leading to this habits? Have any alterations just lately been manufactured on the codebase? Has this situation happened right before below comparable situations? The objective is to narrow down possibilities and detect probable culprits.
Then, take a look at your theories systematically. Try to recreate the challenge inside a controlled natural environment. In case you suspect a particular functionality or part, isolate it and verify if the issue persists. Just like a detective conducting interviews, request your code concerns and Permit the results guide you nearer to the reality.
Spend close awareness to little aspects. Bugs typically hide from the least envisioned spots—like a lacking semicolon, an off-by-1 mistake, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes may possibly disguise the real issue, just for it to resurface afterwards.
Finally, retain notes on Everything more info you tried out and discovered. Equally as detectives log their investigations, documenting your debugging system can preserve time for future challenges and assist Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed problems in intricate devices.
Write Exams
Composing checks is among the most effective approaches to increase your debugging competencies and All round growth performance. Checks don't just help catch bugs early but additionally serve as a security Internet that provides you self esteem when creating adjustments to the codebase. A very well-analyzed software is simpler to debug as it means that you can pinpoint accurately where by and when a problem occurs.
Begin with unit exams, which give attention to personal features or modules. These little, isolated exams can rapidly reveal whether or not a specific bit of logic is Functioning as anticipated. When a test fails, you immediately know where to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily useful for catching regression bugs—issues that reappear just after Earlier getting set.
Next, combine integration exams and finish-to-end assessments into your workflow. These support be sure that different parts of your software operate with each other effortlessly. They’re specially beneficial for catching bugs that occur in advanced programs with numerous factors or products and services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a attribute properly, you require to know its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, producing a failing test that reproduces the bug might be a robust initial step. When the test fails persistently, you could give attention to correcting the bug and watch your check move when The difficulty is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable process—assisting you catch more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s straightforward to become immersed in the situation—gazing your screen for hours, attempting Remedy soon after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The problem from the new point of view.
When you are much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be considerably less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your emphasis. Several developers report obtaining the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during extended debugging periods. Sitting before a display screen, mentally caught, is not only unproductive but will also draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps quickly recognize a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a great general guideline would be to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver about, extend, or do something unrelated to code. It could come to feel counterintuitive, especially beneath limited deadlines, nevertheless it actually contributes to a lot quicker and simpler debugging Ultimately.
In brief, getting breaks isn't an indication of weak spot—it’s a smart tactic. It gives your Mind space to breathe, improves your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Every Bug
Every single bug you come upon is much more than simply A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Completely wrong.
Start by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better methods like unit testing, code critiques, or logging? The answers frequently expose blind spots within your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or preserve a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. Eventually, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial parts of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but individuals that constantly master from their blunders.
Eventually, Every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills will take time, observe, and patience — nevertheless the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.